Self-study 4 + Tutorial 3
This week, I implemented navmesh into my prototype.
Navmeshing was always a scary endeavor to me, so up til now I've always avoided it, but now that I've done it its shockingly simple to get running. The auto-generation was all pretty much flawless, so I could get right into making some enemy creatures.
They are deer, because I like deer.
(Asset is from https://opengameart.org/content/old-deer-male)
That backdrop is a moving obstacle, which you can see in action in the next gif-
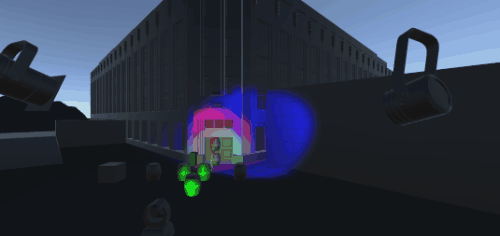
It carves a rectangular prism out of the navmesh, so the deer have to navigate around them.
I set the deer up to spawn on a mouse click, and tweaked the speed to a point where escaping them was difficult, but achievable.
With some stock code, I set up a basic health system and a spawner for the deer.
I also threw together some effects for when the player dies - a death message, a spray of confetti, and a sound effect (a party favour being blown). All of these effects are handled through the unity event system -
- public class PlayerDeathTrigger : MonoBehaviour
- {
- public UnityEvent onPlayerDeath;
- public PlayerHealth player;
- // Start is called before the first frame update
- void Start()
- {
- player.deathActions += onPlayerDeath.Invoke;
- }
- }
- public class PlayerHealth : MonoBehaviour
- {
- ...
- public Action deathActions;
- int health = 0;
- ...
- public void DecreaseHealth(int amount)
- {
- health -= amount;
- SetHealthValueOnUI();
- if (health <= 0)
- {
- deathActions.Invoke();
- }
- }
- }
With these simple scripts, the player's health script receives a list of events to trigger on player death which can all be defined in-engine, through the UI. Simple and extensible- Unity Events save the day once again.
Another fun change- the spotlights now follow the player around, giving some visual interest to the environment. Better yet, the models for the lighting rig move in a (mostly) sensible way to follow the behaviour of the actual lights.
In one last change, the game now has a few health packs hanging around the place, so the player can pick up an extra health point if necessary.
UTAS KIT207 Portfolio
Status | In development |
Category | Other |
Author | ChimeraMusic |
More posts
- Tutorial 5Aug 22, 2022
- Self Study 5Aug 16, 2022
- Self-Study 3 + Tutorial 2Aug 02, 2022
- Test Devlog for HTML code syntaxJul 31, 2022
- Self-study 2 + Tutorial 1Jul 26, 2022
- Week 2 modelling studyJul 19, 2022
Leave a comment
Log in with itch.io to leave a comment.